The steps :
- Download .NET SDK 8
- Install IIS
- Create a directory where our website will live
- Create a new application pool in IIS
- Create a new website
- Create a ASP .NET Core Web App (Razor Pages)
- Install the IIS plug-in module ANCM to deal with Asp .Net Core applications
- Setup in-process deployment in our project
- Publish our application to the website directory
- Create a powershell script to do the deployment for us
The tools needed :
- Powershell core (i’m using 7.4.1)
- Windows (i’m using Windows 11 Home)
Download .NET SDK 8
We can easily install .NET SDK 8 from the following microsoft dotnet official website. After that we can validate it by running the following in the command prompt :
PS C:\Users\joaoc> dotnet --list-sdks
6.0.200 [C:\Program Files\dotnet\sdk]
7.0.405 [C:\Program Files\dotnet\sdk]
8.0.100-rc.2.23502.2 [C:\Program Files\dotnet\sdk]
The version 8 should be our default version, we can check that with the command :
PS C:\Users\joaoc> dotnet --info
.NET SDK:
Version: 8.0.100-rc.2.23502.2
Commit: 0abacfc2b6
Install IIS
Microsoft made it easy for us to install IIS on windows. For that we can go to : Start Menu (press Win Key) -> Type “Windows features” -> Go to “Turn windows features on or off”
After that we should choose “Internet Information Services” and choose at least the following :
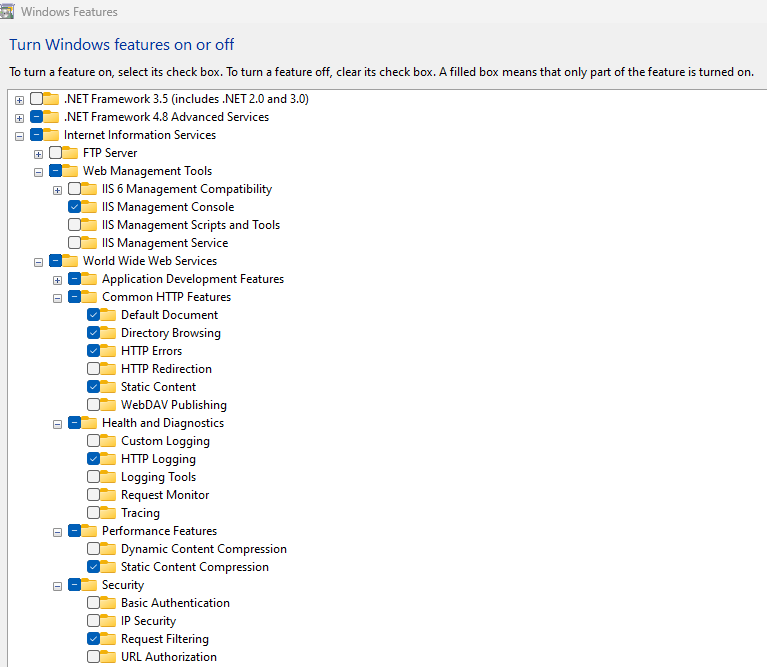
Create a directory for our website
In my case i choose “[your-root]/WebSites/HelloWorldWebSite”. After that, let’s create a simple html file in order to later test or website, by seeing see if the web server can serve us that html file.
Please, take into account that i using powershell core 7.4.1
PS C:\Users\joaoc> cd / ; new-item -type d ./Websites
PS C:\Users\joaoc> cd /WebSites ; new-item -type d ./HelloWorldWebSite
PS C:\Users\joaoc> cd /WebSites/HelloWorldWebSite ; new-item -type f index.html
Let’s put the html in the file :
PS C:\Users\joaoc>notepad++.exe index.html
<h1>
Hello World!
</h1>
Create a new application pool in IIS
To create a new IIS application pool we should open IIS go to “Application Pools”. Hover over “Application Pools”, right-click to open the menu, there we should choose “Add Application Pool”.
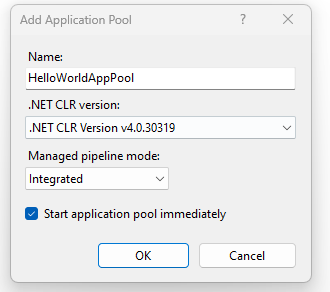
Create a new website
Now, let’s create a website hove over “Sites” and right-click. Choose “Add Website”. We will use the application pool we created before.
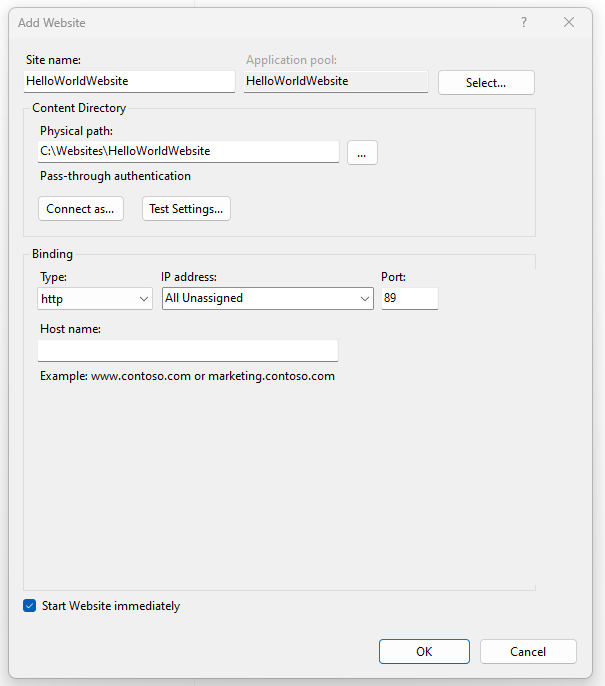
After this we can navigate with our browser to “http://localhost:89” or just test with curl tool :
PS C:\Users\joaoc> curl "http://localhost:89"
Create a ASP .NET Core Web App (Razor Pages)
Let’s go to the dir where we want our app to be and just … create it 🙂 :
# I like to always create sln even thought is not really # necessary
PS {your-dir-path}> dotnet new sln -n hello_world_app
PS {your-dir-path}> dotnet new razor -n hello_world_app -o HelloWorldApp
PS {your-dir-path}> dotnet sln add .\HelloWorldApp\hello_world_app.csproj
Next we will make a small change to our app in the main page :
PS {your-dir-path}> notepad++.exe .\HelloWorldApp\Pages\index.cshtml
Let’s change the <h1> to have “Hello World – V1”.
It should be like the following :
@page
@model IndexModel
@{
ViewData["Title"] = "Home page";
}
<div class="text-center">
<h1 class="display-4">Hello World! - V1</h1>
<p>Learn about <a href="https://learn.microsoft.com/aspnet/core">building Web apps with ASP.NET Core</a>.</p>
</div>
Install the IIS plug-in module ANCM
The ANCM (Asp .Net Core Module ) is a native IIS module that plugs into the IIS pipeline and allow us IIS to deal with .NET Core. We can find it here ASP.NET Core 8.0 Runtime (v8.0.1) – Windows Hosting Bundle.
After installing it we can go to IIS, and in the “Home” view we have something called “Modules” :
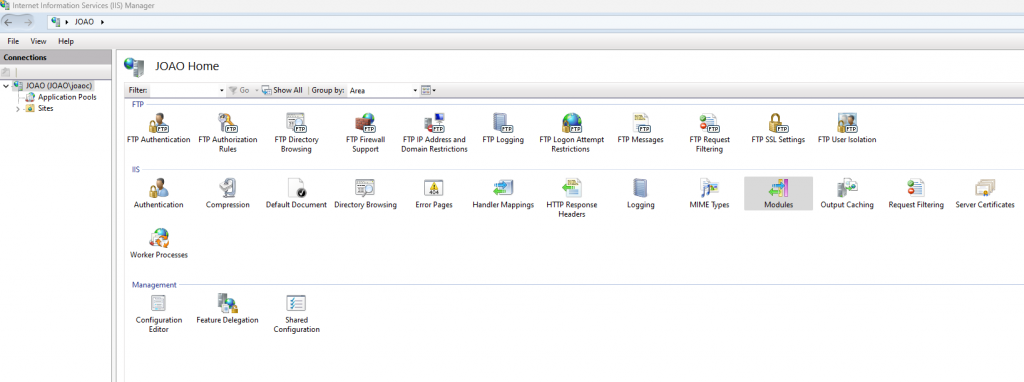
By accessing it, we got the list of IIS modules that constitute the pipeline. We should see the module ANCM each is called in that list “AspNetCoreModuleV2” in these days :
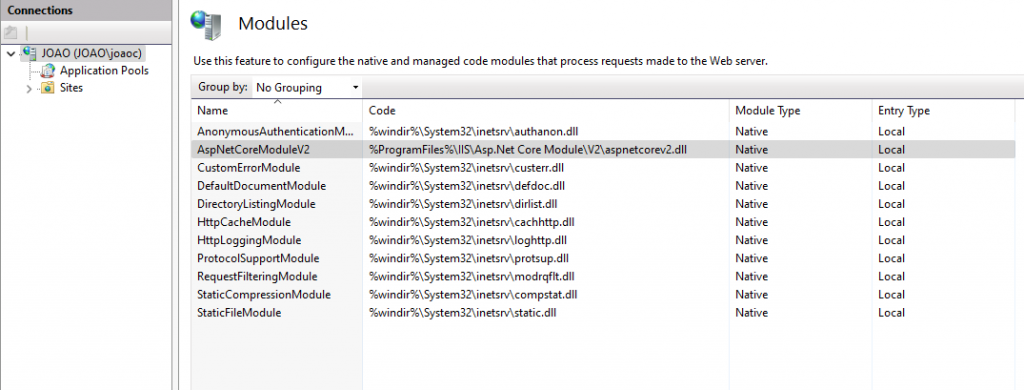
Setup in-process deployment in our project
Now, since we are using IIS as our web server and we want to leverage the in-process model we need to configure our .net core solution for that (If you don’t know what is the in-process model, is something specific for IIS webserver, you can read more about it here). For our case we need to go the .csproj file of our project and add some new properties for the MSBuild.
<PropertyGroup>
<TargetFramework>net8.0</TargetFramework>
<Nullable>enable</Nullable>
<ImplicitUsings>enable</ImplicitUsings>
<AspNetCoreHostingModel>InProcess</AspNetCoreHostingModel>
</PropertyGroup>
That’s it. After this change if we check the web.config file that is generated when building the application in release mode we have :
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<location path="." inheritInChildApplications="false">
<system.webServer>
<handlers>
<add name="aspNetCore" path="*" verb="*" modules="AspNetCoreModuleV2" resourceType="Unspecified" />
</handlers>
<aspNetCore processPath=".\hello_world_app.exe" stdoutLogEnabled="false" stdoutLogFile=".\logs\stdout" hostingModel="InProcess" />
</system.webServer>
</location>
</configuration>
Publish our application to the website director
At this point we have everthing setup, we can navigate to the dir where our IIS website lives and remove the index.html that we created later, we want to publish our razor application there.
# This should be the dir where the sln file is
PS {your-dir-path}> cd HelloWorldApp
PS {your-dir-path}> dotnet publish -c Release -r win-x64 --self-contained false -o "C:\Websites\HelloWorldWebsite"
Now, we can go ahead and check each files we have in our website dir :
PS {your-dir-path}> ls /WebSites/HelloWorldWebsite
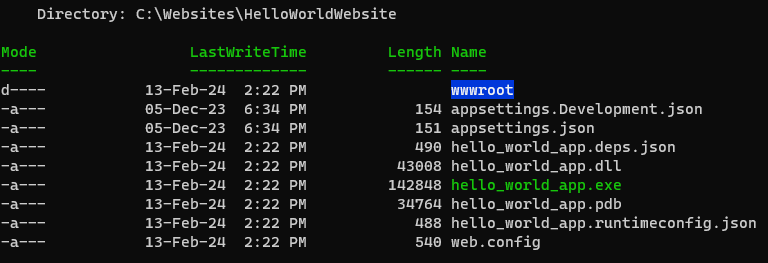
Now we should restart the pool in IIS to make sure the new changes will enter into play.
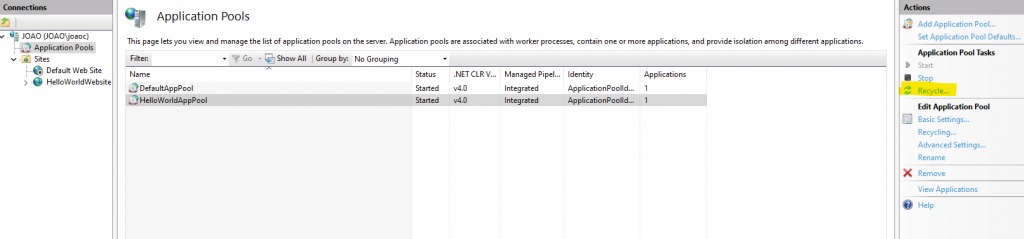
Ok, so let’s see if our just deployed website works by navigating to “http://localhost:89” :
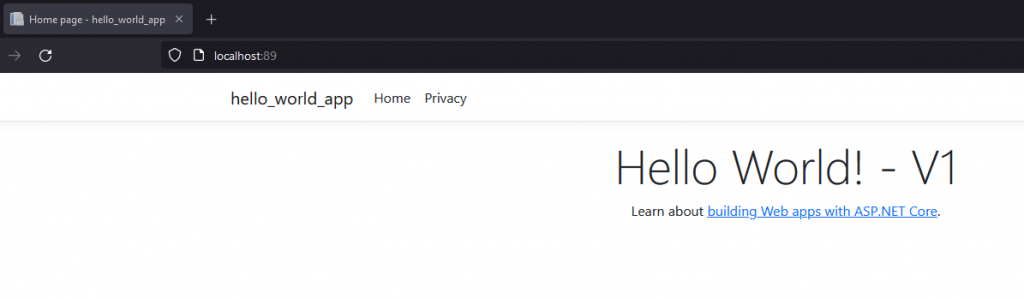
Create a powershell script to do the deployment for us
For the fun of it, let’s create some automation for the deployment. We can do the deployment by using a powershell script for example, each will publish, restart the app pool and the website. This script can be part of the git repo and we can track as well the changes that are made to it.
Maybe something like this ?
# NAME I GIVE TO TE FILE : .\deploy-app-script.ps1
# Define directories
$projectDir = "fill with the path to you csproj"
$websiteDir = "C:\Websites\HelloWorldWebsite"
# Define pool and website names
$appPoolName = "HelloWorldAppPool"
$websiteName = "HelloWorldWebSite"
# Stop pool and website in IIS for deployment
Stop-Website -Name $websiteName -ErrorAction SilentlyContinue | Out-Null
Stop-WebAppPool -Name $appPoolName -ErrorAction SilentlyContinue | Out-Null
Write-Host "Waiting 2 seconds..."
Start-Sleep -Seconds 2
# Remove old files
# carefull with this command ! make sure is exaclty as in # here. This is to remove old artificats from previous # version
Remove-Item -Path $websiteDir\* -Force -Recurse -Confirm:$false
# Set location to the project location
Set-Location -Path $projectDir
dotnet publish -c Release -r win-x64 --self-contained false -o $websiteDir
# Start pool and website in IIS for deployment
Start-WebAppPool -Name $appPoolName -ErrorAction SilentlyContinue | Out-Null
Start-Website -Name $websiteName -ErrorAction SilentlyContinue | Out-Null
However to this we need to install a powershell core module called “WebAdministration” that allow us to manage websites hosted in IIS using powershell core.
> Install-Module WebAdministration
# Import the module to the current session
# usually this is done automtically when using one of the commands
# but if you dont know the commands...
> Import-Module WebAdministration
# to see the version
> Get-Module -Name IISAdministration
# to see all the commands available
> Get-Command -Module IISAdministration
To test this we can change again the title in our website once again :
@page
@model IndexModel
@{
ViewData["Title"] = "Home page";
}
<div class="text-center">
<h1 class="display-4">Hello World! - V2</h1>
<p>Learn about <a href="https://learn.microsoft.com/aspnet/core">building Web apps with ASP.NET Core</a>.</p>
</div>
And run our script :
PS {your-dir-path}> .\deploy-app-script.ps1
Thanks, take care 🙂
Leave a Reply